Πr² Mac OS
I have been given a formula to calculate the Jaccard Coefficient for two real vectors a and b of length n.
It is a conglomoration of NeXTSTEP, A/UX, and Mac OS 9. It is based off the unix kernel and provides compatibility with X11. It features a new theme called Aqua which replaces Platinum from Mac OS 8 and 9. It also adds the Dock as a place to launch applications (Not dissimilar to the underused Launcher) as well as some other features. Yes, writes that appear sequential (to you) can be reordered before being written to disk, primarily because the order seen by your code (or even the OS) may not correspond directly to locations on the disk. Although IDE disks did (at one time) use addressing based on specifying a track.
Is this formula correct? If I calculate the coefficient for the vectors {5, 3, 1, 0, 3} and {7, 1, 3, 2, 1} I get a negative number which I thought is not allowed for metrics).
(5*7 + 3*1 + 1*3 + 0*2 + 3*1) = 44
44 / (12+ 14 - 44) = -22/9
As originally defined by Jaccard, the similarity coefficient is the size of the intersection divided by the size of the union. Since both are sizes, a negative result obviously isn't possible.
What you show in the question looks sort of like the Jaccard similarity for a bit vector. However, for that you need to square each of the terms on the left in the denominator, usually shown something like this:
I suspect the lack of squaring is what's leading to the problem you're currently seeing--without it, we can normally expect the denominator to be negative. More specifically, for one term, (A + B) - (A * B)
to be positive, at least one of A or B must be less than 1.
What is a runtime environment for supposedly “no-overhead” systems languages?
language-agnostic,runtime
C++ requires a few things that aren't required in something like C. For example, it typically involves some overhead for exception handling. Although it may not be strictly required, most systems have at least a tiny bit of a top-level exception handler to tell you that the program shut down..
Primarily Test in O(1)
language-agnostic,primality-test
Yes, all primes are of the form 6k +/- 1, but that doesn't mean that each number that is of the form 6k +/- 1 is prime. Consider 25, which is 6 * 4 + 1. Clearly, 25 is not prime.
What to include in software metric calculation on laravel project?
php,laravel,software-engineering,metrics
It depends on what you're measuring, which is why there's no one answer and similar tools may, or may not, include the vendor folder. If you're interested in the analyzing your own project, then you'll want to point these tools at files you'll be editing. In Laravel 5 that mostly..
Codahale Metrics: using @Timed metrics annotation in plain Java
java,metrics,codahale-metrics
Long story short, you cannot use @Timed without some kind of AOP (be it Spring AOP or AspectJ). A week or two ago, I also decided to add metrics to our project and chose AspectJ for this task (mostly because I used it in the past for similar purpose and..
carbon-tagger does not translate received metrics to Grapthite
metrics,graphite
In a nutshell, correct configuration of described system should looks likes this: That is, statsd/statsdaemon should pass in data to the carbon-relay (or carbon-relay-ng), not to the carbon-cache directly. And carbon-relay will broadcast data to the carbon-tagger and carbon-cache. Also, don't forget that carbon-tagger doesn't work with pickle format, while..
In what kind of code does it makes sense that `typeof NaN` is 'number'
javascript,language-agnostic
In all kinds of code. The constant NaN is explicitly of type 'number'. The value NaN should not be taken to mean what the colloquial phrase 'not a number' means. Instead, it's a specific term from the IEEE floating point spec that describes a family of values whose bit arrangements..
Counting up “broadly”: Not 0,1,2,.,9,10,11,.,99 but 00, 01, 10, 11, 02, 12, 20, 21, 22, 03, ., 99
math,count,language-agnostic,numbers,sequence
So first you want all numbers with the digits 0 in order. i.e. just 00 Then all numbers with the digits 0,1: 00, 01, 10, 11 but excluding 00 Then all numbers with digits 0,1,2: 00, 01, 02, 10, 11, 12, 20, 21, 22 but excluding 00, 01, 10, 11..
Making a consistent API, what's less surprising?
design,architecture,language-agnostic
Case 1: bar() also panic when hasBar() is false This will not be surprising behavior to me as if I am not assigning anything to a float, I shouldn't be expecting anything in return form it. Returning NaN is a convenient way to avoid exception in program and letting it..
How to find largest square of palindrome in a matrix
algorithm,language-agnostic,dynamic-programming
Assuming that you can solve the following problem: Ending at cell (i, j) is there any palindrome with different length horizontally and vertically. Hint for above problem: boolean[][][]palindrome;//Is there any palindrome ending at (i , j) has length k for(int i = 0; i < n; i++){ for(int j =..
spring boot repository metrics
spring,spring-boot,metrics
In order to access all Public Metrics printed by the Spring Boot actuator framework you need to inject a 'Collection' into your component and then access it to read all the metrics. Here is an example: import org.springframework.beans.factory.annotation.Autowired; import org.springframework.boot.actuate.endpoint.PublicMetrics; import org.springframework.boot.actuate.metrics.Metric; import org.springframework.stereotype.Component; import java.util.Collection; @Component public class ActuatorMetricsPrinter..
What is the cleanest way to round a value to nearest 45 degrees?
language-agnostic,rounding
I'd divide the value by 45°, round, and then multiply back by 45°. Pseudocode: deg = round(deg / 45) * 45; Assumption: the / operator returns a floating-point representation and does not perform integer division. If the specific language does not treat it like this it should be handled explicitly..
What's the algorithm to assign people their most preferred value based on a list of their top choices?
algorithm,sorting,language-agnostic,ranking,choice
This is the Assignment problem. You could use, for instance, the Hungarian Algorithm. You just need to come up with a way to turn the user/player preferences into costs. Perhaps when a person gets their first choice, the cost is -3, second choice is -2, third choice is -1, etc..
Is there any formula that maps an int on the range [0.πR²] to the (x,y) coordinates inside the circle of radius R?
algorithm,math,language-agnostic,functional-programming,formula
Note that the most circles with radius R contain less than πR² integer points within the boundary, so usually that map doesn't exist. Reference: Gauss circle problem And I suspect that even for good R values there is no simple formula, only iterative aprroach (look at (1) formula in the..
How to find the endpoint of a vector giving its length and startpoint
math,vector,language-agnostic,geometry
Normalize direction vector, if needed: Len = Sqrt( dirx^2 + diry^2 ) dx = dirx / Len dy = diry / Len then find endpoint: end_x = x0 + 40 * dx end_y = y0 + 40 * dy ..
Why sonar metrics are sometimes wrong?
c++,sonarqube,metrics,sonarqube-4.5
With an isolation of the specific problematic files, I found parsing errors : com.sonar.sslr.api.RecognitionException: Parse error at line 123 column 0 failed to match all of: parametersAndQualifiers virtSpecifier ctorInitializer compoundStatement functionTryBlock parametersAndQualifiers bracedInitList It appears sonar does not support some boost instructions like this one : BOOST_CLASS_VERSION(MyClass, 0) So next..
Golang: implements http server health checking. gocraft/health
go,http-headers,metrics,endpoints,endpointbehavior
Finnaly I chosed 'gocraft/health' great library example of using import ( 'github.com/gocraft/health' ) //should be gloubal Var var stream = health.NewStream() func main() { // Log to stdout! stream.AddSink(&health.WriterSink{os.Stdout}) // Make sink and add it to stream sink := health.NewJsonPollingSink(time.Minute*5, time.Minute*20) stream.AddSink(sink) // Start the HTTP server! This will expose..
How to find m in c = m^e (mod n) if c, e, n are known
language-agnostic,rsa,number-theory,modular-arithmetic
Well, sort of.. Suppose that you have determined the number 'd' such that d*e=1 (mod phi(n)) Where phi(n) is the size of the set of relatively prime numbers relative to n. For example, if n=pq where p and q are prime, then phi(n)=(p-1)*(q-1). Then m=c^d (mod n) In the case..
Alternative to multiple or statements in an if block in Javascript
javascript,language-agnostic
matches = ['An', 'Apple', 'A', 'Day']; if (matches.indexOf(x) > -1){ return true; } else{ return false; } ..
Algorithm to determine an order of processing/sorting
algorithm,sorting,language-agnostic,order,priority
Perhaps you could use a legal-style paragraph numbering.: 1, 1.12, 1.12.1, 1.12.2, 1.12.2.5 and so on. You can always insert a new entity between existing entities by extending to a new level of '.1'. Quite how you stop users assigning absurdly high priorities to otherwise routine documents is another problem..
Consistency guarantee of file system regarding sequential write
io,language-agnostic,consistency,os-agnostic
Yes, writes that appear sequential (to you) can be reordered before being written to disk, primarily because the order seen by your code (or even the OS) may not correspond directly to locations on the disk. Although IDE disks did (at one time) use addressing based on specifying a track,..
OpenShift Metrics Cartridge
openshift,metrics
No, that cartridge is no longer available. You should try running something like New Relic or a similar service instead.
Hypothetical Non-Null Language - How would a Linked List be implemented?
null,linked-list,language-agnostic,programming-languages,non-nullable
One solution could be using something that is equivalent to the Maybe type. It has either the value Just x, where x in this case is the next node in the list, or Nothing. The Maybe monad is best demonstrated in Haskell. http://learnyouahaskell.com/a-fistful-of-monads..
Calculate distance on a grid between 2 points
java,math,language-agnostic,distance
This is pretty simple: You move diagonally towards the goal until you're either on the same row or same col. This will be min(dx, dy) steps. Let's call this d (for diagonal steps) Then you move on a straight line towards the goal. This will be max(dx, dy) - d..
Undirected, weighted graph partitioning
algorithm,language-agnostic,graph-algorithm
What you are looking for is called the minimum cut of a graph. By the Max-flow_min-cut_theorem the value of the minimum cut is equal the the value of the maximum flow. Computing the maximum flow in a network is a standard problem and there are several algorithms for computing this..
Greedy algorithm: highest value first vs earliest deadline first
algorithm,language-agnostic,job-scheduling,greedy
In fact, neither 'earliest deadline first', 'highest profit first' nor 'highest profit/duration first' are correct algorithm.. Assume 2 jobs: Job A has profit 1, time duration 1, deadline before day 1; Job B has profit 2, time duration 2, deadline before day 2; Then 'earliest deadline first' fails to get..
Bracket and brace usage in BNF?
syntax,language-agnostic,grammar,bnf,ebnf
Braces and brackets are EBNF constructs -- the E stands for extended. Simple BNF is just a context-free grammar with no extra syntactic sugar for recursive things, so you have to write it out with a nested recursive rule: <function parameter list> ::= ( <param-list> ) ( ) <param-list>..
Convert between UTF:s without intermediate encoding
algorithm,language-agnostic,utf
I think the question is more interesting than the comments suggest: UTF-8 -> UTF-16: It's not possible if you write UTF-16 word by word (if you write byte by byte, I think it depends on UTF-16LE vs. UTF-16BE). UTF-8 encodes 7 bit with 1 byte, 11 bit with 2 bytes..
Iterator to produce unique random order?
language-agnostic,iterator,random-sample
Encryption is reversible, hence an encryption is a one-to-one mapping from a set onto itself. Pick a block cypher with a large enough block size to cover the number of items you have. Encrypt the numbers 0, 1, 2, 3, 4, .. This will give you a non-repeating ordered list..
Origin of inheritance in programming languages
oop,language-agnostic
The first object oriented language was Simula. Specifically Simula67, introduced in 1967 and implemented in 1968. Simula67 added the concept of classes and subclasses (inheritance) inspired by a research paper (theory, not any specific language) by C.A.R. Hoare describing the concept of classes as a collection of data in memory..
Is Swift the only (mainstream) language with overflow checking arithmetic?
performance,swift,language-agnostic,arithmetic-expressions
Performance There's necessarily a cost to that kind of verification. At the lowest level, the operation which causes the overflow takes a single CPU operation. Checking that there was or not and overflow during this operation requires at least one more operation (e.g. using jump operations (branching) which consider the..
Where is the Balance Between Dependency Injection and Abstraction?
java,oop,design-patterns,dependency-injection,language-agnostic
The point with Dependency Injection and more specifically the Dependency Inversion Principle is that you don't want all types in your application to depend on other concrete types. Not only does this hinder testability, but also maintainability and flexibility. No matter what you do, and no matter how many abstractions..
gethostbyname and endianness - how are the bytes returned?
winapi,language-agnostic,winsock,endianness
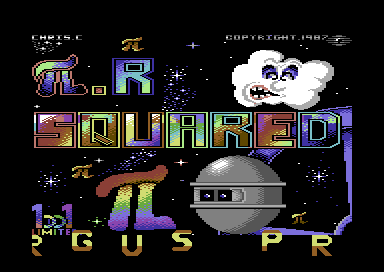
The addresses contained in the hostent structure are in network byte order. If you have code that suggests otherwise then you are misinterpreting that code and reaching the wrong conclusion. In network byte order, on a little endian host, 127.0.0.1 is 0x0100007f. To see how this works, remember that on..
Turn an ascii character code to a value between 0-26
Adobe photoshop cs3 authorization code generator for mac. algorithm,language-agnostic,ascii
The following code should work in C and C++: int codeFor(char ch) { if (ch >= 'A' && ch <= 'Z') { return ch - 'A'; } else if (ch >= 'a' && ch <= 'z') { return ch - 'a'; } else { /* do something to report an..
Graphite whisper losing old data
metrics,graphite,statsd,grafana,whisper
I found the issue. Using whisper-info.py, the retention times were shown to be 7 days on all the old metrics, as I was witnessing. Newer metrics were being retained just fine. The current storage-schemas.conf didn't have 7 days specified anywhere.. but the previous storage-schemas.conf file could have had it. It..
Sublinear algorithm to find the maximum contiguous range with elements >= k in an array
algorithm,language-agnostic
Yes, it is possible. You can use a special data structure that can be constructed in linear time and answers a range maximum query in O(1). It is rather complex, you can read about it here. When we have this data structure, we can use binary search to find the..
Optimal Compare Algorithm for finding string matches in List of strings C#
c#,string,algorithm,optimization,language-agnostic
I decided to add this answer not because it is the optimal solution to your problem, but to illustrate two possible solutions that are relatively simple and that are somewhat in line with the approach you seem to be following yourself. The (non-optimized) sample below provides an extremely simple prefix..
Pass parameters between method name
methods,language-agnostic,parameter-passing
Of course, there is Smalltalk which is really expressive with its keyword messages.. n := collection size // 2. head := collection copyFrom: 1 to: n. Other than that, you will find that in ADA or Python, and probably some others you can prefix each argument with a key (which..
What do “key buffer size” and “total MyISAM indexes” unit sizes mean?
mysql,myisam,metrics,mysqltuner
Are you sure you looked into the documentation. Quoting From MySQL Documentation: To minimize disk I/O, the MyISAM storage engine employs a cache mechanism to keep the most frequently accessed table blocks in memory: For index blocks, a special structure called the key cache (or key buffer) is maintained. To..
How to handle exceptions during logging?
c#,exception,logging,exception-handling,language-agnostic
Better use a logging framework like Log4Net. Also, there is a very small chance of running into exceptions in using the TextWriter class. You just have to make sure that: Your paths and permissions are correct when opening the stream/TextWriter in the first place. Your TextWriter object is not Disposed..
Cassandra read latencies
cassandra,metrics,latency
The ClientRequest latency is as coordinator, possibly including network latencies between the cluster nodes. The ColumnFamily metrics are local only so its the time to write to memtable/commitlog or pull the data from the sstables.
Is it good practice to use magic numbers?
html,sql,language-agnostic,coding-style
ID numbers are generally assigned arbitrarily by the database (e.g. with MySQL AUTO_INCREMENT), and they're just accidents of timing. It's generally a bad idea to depend on them and hard-code them into your code. The corresponding name is less likely to change, so if you have to hard-code something, that's..
Jaccard Coefficient
language-agnostic,metrics,coefficients
As originally defined by Jaccard, the similarity coefficient is the size of the intersection divided by the size of the union. Since both are sizes, a negative result obviously isn't possible. What you show in the question looks sort of like the Jaccard similarity for a bit vector. However, for..
Why ever use CachedGauage in Dropwizard Metrics?
java,metrics,dropwizard,codahale-metrics Betsafe casino mobile.
Quoting the same link you added: Cached Gauges A cached gauge allows for a more efficient reporting of values which are expensive to calculate What if your metric takes around two seconds seconds to calculate, or even minutes? Would you calculate every time the user request the data? Makes sense..
Average Cyclomatic Complexity of multiple files
static-analysis,metrics,code-metrics,cyclomatic-complexity Minecraft pc gamer demo crack.
Cyclomatic complexity in effect measures the number of decisions in your source code. (Its actually more complex than that in general, but decays to that in the case of structured code). It is often computed as #decisions+1, even in the more complex case (yes, that's an approximiation). So, if you..
Mac Os Download
Prevent SQL injection when building query
sql-server,language-agnostic
Mac Os Catalina
This is not specific to any one DB API. TL;DR: Don't pass 'SQL fragments' around. Rather than passing complete clauses fro a select statement, or (sub-)expressions to add into a select clause, pass the components keeping the user data separate from the identifiers. In this case do not pass name..
How do define a metric in order to evaluate programmers? [closed]
There is probably no standard way to deal with this. Because 1. there is no standard definition of which programer is the best, and if there were something close, 2. it would probably not rely on those metrics. So you are left with subjective choices here. As mentioned in the..
C - What should scripts do in programs [closed]
c,scripting,lua,language-agnostic,scripting-language
Πr2 Mac Os Downloads
They should interact with the various 'programming primitives' that the native code implements. That is, the native code should only do enough to allow the scripts to function within the game (although 'function' can sometimes mean speed-wise). If that sounds cyclical.. it is. There's no complete way to define at..
Icosahedron joining edge angles
math,language-agnostic
Πr2 Mac Os Catalina
I think what you want is the dihedral angle. These are well know and you can see a table at https://en.wikipedia.org/wiki/Table_of_polyhedron_dihedral_angles . For an Icosahedron the angle is π − arccos(√5/3) or approximately 138.19°. The angle in your diagram is 360° - 138.19° - 90° - 90° = 41.81°.
How many times is x=x+1 executed in theta notation in terms of n?
algorithm,language-agnostic,time-complexity,complexity-theory,analysis
Answering for the first part :- for i = 1 to 526 for j = 1 to n^2(lgn)^3 for k = 1 to n x=x+1 This program will run 526 * n^2 * (lg n)^3 * n times = 526 * n^3 * (lg n)^3 times. So, x = x..